As XF documentations say, your platform-application (Droid, iOS or WP) should call Forms.Init() at startup.
That should be done:
-
In iOS: in the AppDelegate.FinishedLaunching method
- global::Xamarin.Forms.Forms.Init();
-
In Droid: in the MainActivity. OnCreate (Bundle bundle)
- global::Xamarin.Forms.Forms.Init(this, bundle);
-
In Win Phone: in your application's MainPage constructor
- global::Xamarin.Forms.Forms.Init();
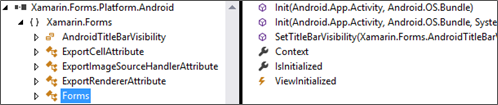
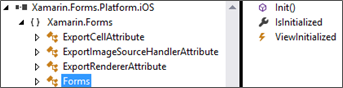
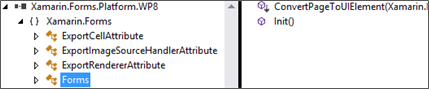
What does Forms.Init do?
On droid
Here is an example of what Forms.Init method does on Android:
public static void Init(Activity activity, Bundle bundle)
{
// get the calling assembly
Assembly callingAssembly = Assembly.GetCallingAssembly();
// Call SetupInit
SetupInit(activity, callingAssembly);
}
Let us continue following the call to SetupInit():
private static void SetupInit(Activity activity, Assembly resourceAssembly)
{
// set the Context to current activity
Context = activity;
// initialize resources for this assembly
ResourceManager.Init(resourceAssembly);
// set the AccentColor according to OS version
if (Build.VERSION.SdkInt <= BuildVersionCodes.GingerbreadMr1)
{
Color.Accent = Color.FromHex("#fffeaa0c");
}
else
{
Color.Accent = Color.FromHex("#ff33b5e5");
}
// log (if not initialized)
if (!IsInitialized)
{
Log.get_Listeners().Add(new DelegateLogListener(
(c, m) => Trace.WriteLine(m, c)));
}
// set the Device.OS version and platform services (here android)
Device.OS = TargetPlatform.Android;
Device.PlatformServices = new AndroidPlatformServices();
// recreate the device info from this activity
if (Device.info != null)
{
((AndroidDeviceInfo) Device.info).Dispose();
Device.info = null;
}
IDeviceInfoProvider formsActivity = activity as IDeviceInfoProvider;
if (formsActivity != null)
{
Device.Info = new AndroidDeviceInfo(formsActivity);
}
// recreate the ticker
AndroidTicker ticker = Ticker.Default as AndroidTicker;
if (ticker != null)
{
ticker.Dispose();
}
Ticker.Default = new AndroidTicker();
// initialize renderers (if not initialized (again))
if (!IsInitialized)
{
// register renderers for attributes export / cell / image source
Type[] typeArray1 = new Type[]
{ typeof(ExportRendererAttribute),
typeof(ExportCellAttribute),
typeof(ExportImageSourceHandlerAttribute)
};
// registrer the handlers of these types
Registrar.RegisterAll(typeArray1);
}
// set the device idiom according to screen width dpi
Device.Idiom = (Context.Resources.Configuration.SmallestScreenWidthDp >= 600)
? TargetIdiom.Tablet : TargetIdiom.Phone;
// set search expression default (if not already set)
if (ExpressionSearch.Default == null)
{
ExpressionSearch.Default = new AndroidExpressionSearch();
}
// set initialzed flag
IsInitialized = true;
}
On iOS
public static void Init()
{
if (!IsInitialized)
{
// set initialized flag
IsInitialized = true;
// set the AccentColor
Color.Accent = Color.FromRgba(50, 0x4f, 0x85, 0xff);
Log.get_Listeners().Add(new DelegateLogListener(
// obscure decompilation of an anonymous methodJ
<>c.<>9__9_0
?? (<>c.<>9__9_0 = new Action<string, string> (<>c.<>9.<Init>b__9_0))));
// device os and platform services
Device.OS = TargetPlatform.iOS;
Device.PlatformServices = new IOSPlatformServices();
Device.Info = new IOSDeviceInfo();
// set the ticker
Ticker.Default = new CADisplayLinkTicker();
// register renderers for attributes export / cell / image source
Type[] typeArray1 = new Type[]
{ typeof(ExportRendererAttribute),
typeof(ExportCellAttribute),
typeof(ExportImageSourceHandlerAttribute)
};
Registrar.RegisterAll(typeArray1);
// set device idiom
Device.Idiom = (UIDevice.get_CurrentDevice ().get_UserInterfaceIdiom() == 1L)
? TargetIdiom.Tablet : TargetIdiom.Phone;
// set search expression default
ExpressionSearch.Default = new iOSExpressionSearch();
}
}
On Win Phone
public static void Init()
{
if (!isInitialized)
{
// create an event trigger object (why?)
// note: constructor initializes an EventNameProperty DependencyProperty
new EventTrigger();
string name = Assembly.GetExecutingAssembly().GetName().Name;
ResourceDictionary dictionary1 = new ResourceDictionary();
// load xaml resources
dictionary1.set_Source(
new Uri(string.Format("/{0};component/WPResources.xaml", name),
UriKind.Relative));
// add resources to merged dictionaries
Application.get_Current().get_Resources().get_MergedDictionaries().Add(dictionary1);
// set accent( color from resources
Color color = (Application.get_Current().get_Resources().get_Item("PhoneAccentBrush") as SolidColorBrush).get_Color();
byte introduced3 = color.get_R();
byte introduced4 = color.get_G();
byte introduced5 = color.get_B();
Color.Accent = Color.FromRgba((int)introduced3,
(int)introduced4,
(int)introduced5,
(int)color.get_A());
// log
Log.get_Listeners().Add(new DelegateLogListener(<> c.<> 9__3_0
?? (<> c.<> 9__3_0 = new Action<string,
string>(<> c.<> 9.< Init > b__3_0))));
// set device os and platform services
Device.OS = TargetPlatform.WinPhone;
Device.PlatformServices = new WP8PlatformServices();
Device.Info = new WP8DeviceInfo();
// register renderers for attributes export / cell / image source
Type[] typeArray1 = new Type[]
{ typeof(ExportRendererAttribute),
typeof(ExportCellAttribute),
typeof(ExportImageSourceHandlerAttribute)
};
Registrar.RegisterAll(typeArray1);
// set ticker default
Ticker.Default = new WinPhoneTicker();
// set device idiom
Device.Idiom = TargetIdiom.Phone;
// set search expression default
ExpressionSearch.Default = new WinPhoneExpressionSearch();
// set initialzed flag
isInitialized = true;
}
}